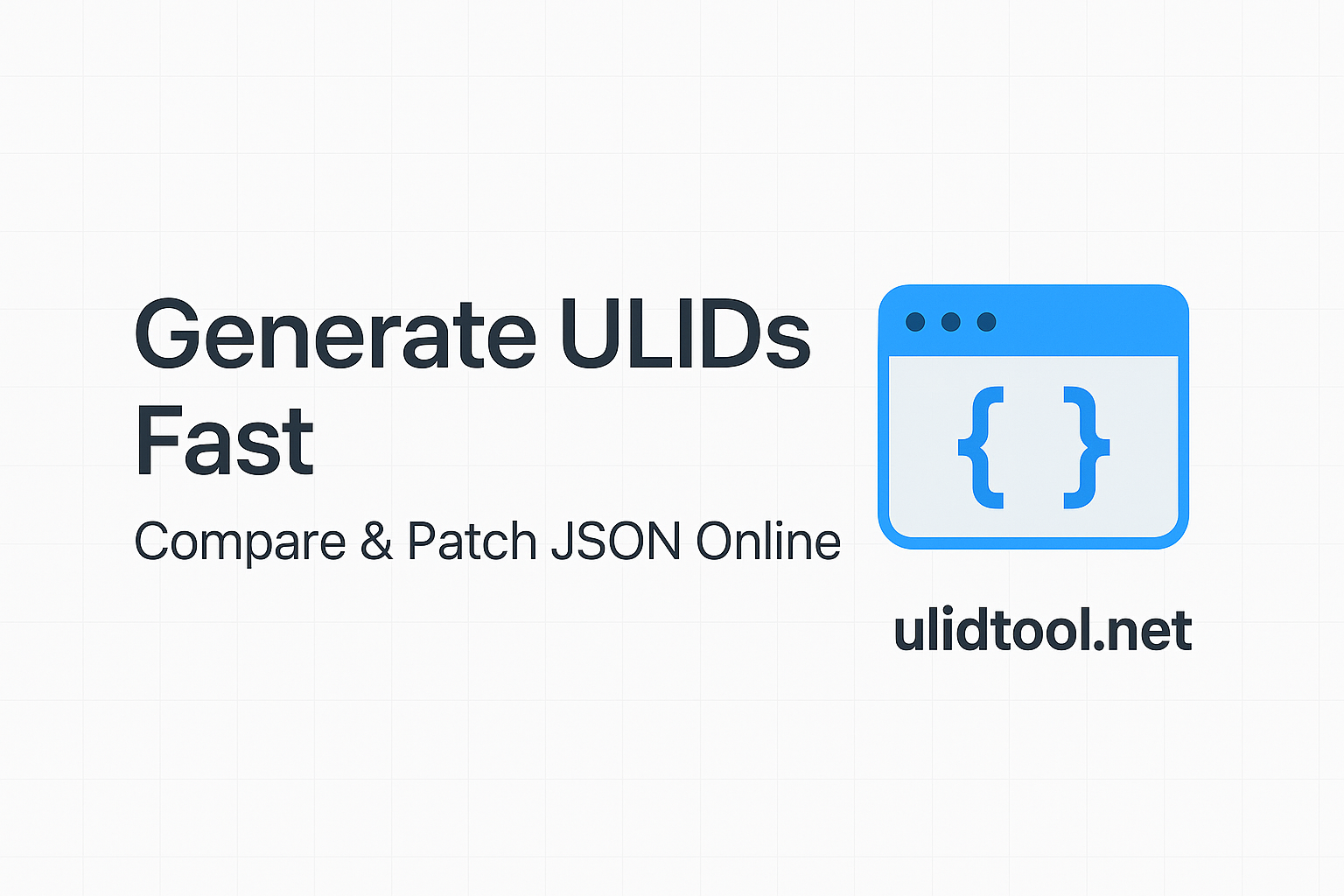
生成 ULID
为现代应用程序提供快速、高效和可排序的标识符
所有数据都保留在您的浏览器中。不会发送到服务器。
Options
ULID 与 UUID 比较
为什么在许多情况下 ULID 比 UUID 更好?
ULID
推荐时间可排序
前 10 个字节是自然排序的 Unix 时间
Crockford 的 Base32
更高效的编码,不区分大小写,没有特殊字符
单调递增选项
可以为相同时间戳生成单调递增的值
人类可读
在日志和数据库中更容易调试和阅读
ULID 示例:
UUID
不可按时间排序
随机字节不提供任何排序保证
十六进制编码
效率较低,相同信息需要更多字符
无单调性
没有内置机制用于单调递增值
可读性较差
在日志和数据库中更难区分和识别
UUID 示例:
性能比较
代码示例
以下是一些代码示例,帮助您开始
// Node.js / 浏览器
// 安装: npm install @ulid/javascript
import { ulid } from '@ulid/javascript';
// 生成新的 ULID
const myUlid = ulid(); // "01F8MECHZX3TBDSZ7XR8H8J1R4"
// 使用自定义时间戳创建 ULID (毫秒)
const customTimestamp = 1616239022000;
const ulidWithCustomTime = ulid(customTimestamp);
// 创建单调递增的 ULID (用于同一毫秒内的多个 ID)
import { monotonicFactory } from '@ulid/javascript';
const monotonic = monotonicFactory();
const ulid1 = monotonic(); // "01F8MECHZX3TBDSZ7XR8H8J1R4"
const ulid2 = monotonic(); // "01F8MECHZX3TBDSZ7XR8H8J1R5" (递增)
// 从 ULID 提取时间戳
const timestamp = ulid.decode(myUlid).time;
console.log(new Date(timestamp).toISOString());
JavaScript: npm install @ulid/javascript
解码 ULID
从 ULID 中提取时间戳和随机性
Validation Results
UUID / ULID 转换器
在 UUID 和 ULID 格式之间转换
注意:将 UUID 转换为 ULID 时,将使用当前时间戳。这意味着同一个 UUID 在不同时间会产生不同的 ULID。
ULID Comparator
比较结果
组件分析
Entropy Difference
Character-by-Character Diff
Timestamp Part
Random Part
Detailed Diff View
Position | |
First ULID | |
Second ULID | |
Difference |
时间戳转换器
转换结果
JSON 工具
用于处理 JSON 数据的实用工具
JSONPath 测试器
Test and validate JSONPath expressions against your JSON data.
JSON Patch
Apply RFC 6902 JSON Patch operations to transform JSON documents.
JSON Patch 生成器
Generate JSON Patch documents by comparing two JSON objects.
JSON 差异比较
Compare two JSON objects and visualize the differences in multiple formats.
Test and validate JSONPath expressions against your JSON data.
JSON Data
JSONPath Expression
Result
JSONPath Reference
Basic Syntax
Symbol | Description |
---|---|
$ | Root object |
@ | Current object |
. | Child operator |
.. | Recursive descent |
* | Wildcard. All objects/elements |
[] | Subscript operator |
[,] | Union operator |
Examples
JSONPath | Description |
---|---|
$.store.book[0] | First book |
$.store.book[-1] | Last book |
$.store.book[0,1] | First two books |
$.store.book[*] | All books |
$.store.book[*].author | All authors |
$.store.book[?(@.price < 10)] | Books less than 10 dollars |
$..book[?(@.isbn)] | All books with ISBN |
Generate JSON Patch documents by comparing two JSON objects.
Source JSON
Target JSON
Generated JSON Patch
Verification
JSON Patch Operations (RFC 6902)
Operation | Description |
---|---|
add | Adds a value to an object or inserts it into an array |
remove | Removes a value from an object or array |
replace | Replaces a value |
Operation | Description |
---|---|
move | Moves a value from one location to another |
copy | Copies a value from one location to another |
test | Tests that a value at the target location is equal to a specified value |